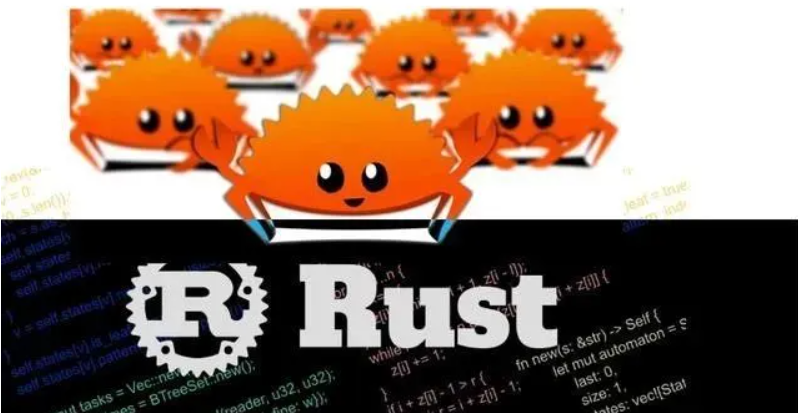
Rust Study Day1
本文最后更新于 2024-03-29,本文发布时间距今超过 90 天, 文章内容可能已经过时。最新内容请以官方内容为准
Rust Study Day1
1. Hello, world!
Whatever you do, you must start with a hello world.
Recommand use cargo new
to create a new project.
So, let’s create a new project.
Type the following command in your vscode terminal:
cargo new hello_world_rust
cd hello_world_rust
code .
Now, you can see the project in your vscode navigator located on the left.
1.2. Run Hello, world!
Open the src/main.rs
file.
// main.rs
fn main() {
println!("Hello, world!");
}
Let’s run the project.
Type the following command in your vscode terminal:
cargo run
You will see the “hello world!” in your terminal.
2. Variables, functions and process controls
2.1. Variables
- Variables are used to store data.
- Variables are declared using the
let
keyword. - Variables are immutable.
- If you want to change the value of a variable, you must re-declare it or use the
mut
keyword. - And rust can auto infer the type of the variable. Like C#
[var]
.
Some examples:
let a = 1;
let b = 2;
a =3; // Ops, error! a is immutable!
let mut c = 3;
c = 4; // Ok, c is mutable!
2.2. Functions
- Functions are used to perform a specific task.
- functions are declared using the
fn
keyword. - Different functions can have a different prefix type. Such as:
pub
,pub(crate)
,etc …
pub mod funtions; looks seem as use crate::math_functions;
Some examples:
// math_functions.rs
fn add(a: i32, b: i32) -> i32 {
a + b
return a + b;
}
pub fn substract(a: i32, b: i32) -> i32 {
a - b
return a - b;
}
// main.rs
use crate::math_functions;
fn main() {
let a = 1;
let b = 2;
println!("add: {}", add(a, b)); // Ops! Fault! Due to the add function is private in the math_functions.rs file.
println!("substract: {}", math_functions::substract(a, b)); // Ok! substract is public in the math_functions.rs file. And the crate keyword is used to import the math_functions.rs file.
more info for Rust Functions and Rust Module
CHINESE: Rust 程序设计语言 中文版
2.3. Process controls
- Rust has a powerful control flow mechanism.
- Rust has 3 control flow mechanisms:
if
,for
,while
,loop
,match
…
// if
if number < 5 {
println!("condition was true");
} else if number > 8 {
println!("condition was false");
} else {
println!("condition was neither true nor false");
}
// match
let match_num = 10;
match match_num {
1 => println!("match_num is 1"),
2 => println!("match_num is 2"),
10 => println!("match_num is 10"),
_ => println!("match_num is not 10"),
}
// for
// from 1 to 9, left close, right open
// but for i in 1..=10, it from 1 to 10. left close, right close
for number in 1..10 {
println!("the number is: {}", number);
if number % 2 == 0 {
println!("the number is even");
break;
}
}
// iterator
for ele in [1, 2, 3, 4, 5] {
println!("the element is: {}", ele);
}
// while
let mut while_num = 1;
while while_num < 10 {
println!("the number is: {}", while_num);
while_num += 1;
if while_num % 2 == 0 {
println!("the number is even");
break;
}
}
// loop
let mut loop_num = 1;
loop {
println!("the number is: {}", loop_num);
loop_num += 1;
if loop_num % 2 == 0 {
println!("the number is even");
break;
}
}
What’s the difference between if
and match
?
if
is a conditional statement, andmatch
is a pattern matching statement.
Day Day Conclusion
- Need to learn more about rust. Such as:
- use crate::[m];
- pub mod [m];
- Rust is a mutable and imutable language.
本文是原创文章,采用 CC BY-NC-ND 4.0 协议,完整转载请注明来自 Unic
评论
匿名评论
隐私政策
你无需删除空行,直接评论以获取最佳展示效果